文章目录
- 探索Python文本处理的瑞士军刀:re库
- 第一部分:背景介绍
- 第二部分:`re`库是什么?
- 第三部分:如何安装`re`库?
- 第四部分:`re`库函数使用方法
- 1. `re.search(pattern, string)`
- 2. `re.match(pattern, string)`
- 3. `re.findall(pattern, string)`
- 4. `re.sub(pattern, repl, string)`
- 5. `re.split(pattern, string)`
- 第五部分:场景应用
- 场景1:验证邮箱地址
- 场景2:提取HTML标签
- 场景3:替换文本中的数字
- 场景4:分割字符串
- 场景5:查找所有IP地址
- 第六部分:常见Bug及解决方案
- Bug1:模式不匹配
- Bug2:特殊字符未转义
- Bug3:模式匹配失败
- 第七部分:总结
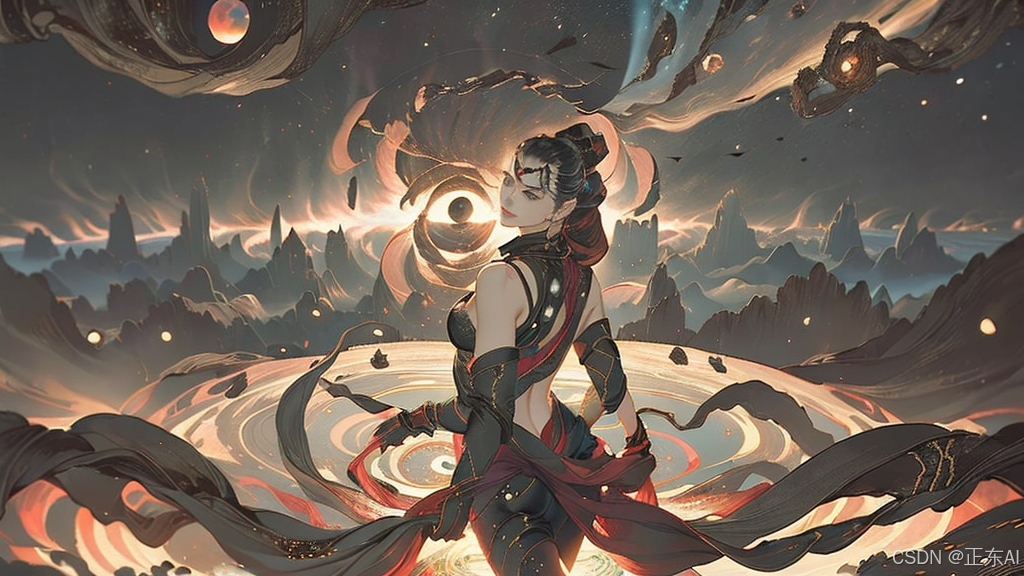
探索Python文本处理的瑞士军刀:re库
第一部分:背景介绍
在Python的世界里,处理字符串是一项常见的任务。无论是数据清洗、日志分析还是网页爬虫,我们经常需要对字符串进行复杂的操作。这时候,一个强大的工具库就显得尤为重要。re
库,作为Python的标准库之一,提供了正则表达式的支持,使得文本处理变得异常强大和灵活。它能够识别字符串中的模式,执行搜索、替换、分割等操作,是任何Python开发者工具箱中的必备工具。
第二部分:re
库是什么?
re
是Python中用于处理正则表达式的库。正则表达式是一种文本模式,包括普通字符(例如,字母a到z)和特殊字符(称为"元字符")。re
库允许你编译正则表达式模式,然后使用这些模式匹配字符串、替换匹配的子串、查找匹配的位置等。
第三部分:如何安装re
库?
由于re
是Python的标准库,你不需要额外安装它。只需在Python脚本中导入即可使用:
import re
第四部分:re
库函数使用方法
1. re.search(pattern, string)
搜索字符串中第一次出现的模式。
match = re.search(r'\d+', 'Hello 123 world')
if match:print(match.group()) # 输出:123
2. re.match(pattern, string)
从字符串的开始位置匹配模式,如果匹配失败则返回None。
match = re.match(r'Hello', 'Hello world')
if match:print(match.group()) # 输出:Hello
3. re.findall(pattern, string)
找出字符串中所有匹配的子串,并返回一个列表。
matches = re.findall(r'\d+', 'abc 123 def 456')
print(matches) # 输出:['123', '456']
4. re.sub(pattern, repl, string)
替换字符串中匹配的子串。
new_string = re.sub(r'\d+', 'number', 'abc 123 def 456')
print(new_string) # 输出:abc number def number
5. re.split(pattern, string)
按照匹配的模式分割字符串。
parts = re.split(r'\s+', 'one two three four')
print(parts) # 输出:['one', 'two', 'three', 'four']
第五部分:场景应用
场景1:验证邮箱地址
email = "user@example.com"
if re.match(r"[^@]+@[^@]+\.[^@]+", email):print("有效的邮箱地址")
else:print("无效的邮箱地址")
场景2:提取HTML标签
html = "<html><head><title>Test</title></head><body><p>Paragraph</p></body></html>"
tags = re.findall(r'<[^>]+>', html)
print(tags) # 输出:['<html>', '<head>', '<title>Test</title>', '</head>', '<body>', '<p>Paragraph</p>', '</body>', '</html>']
场景3:替换文本中的数字
text = "I have 3 apples and 5 oranges."
new_text = re.sub(r'\d+', 'many', text)
print(new_text) # 输出:I have many apples and many oranges.
场景4:分割字符串
sentence = "one, two, three, four"
words = re.split(r',\s*', sentence)
print(words) # 输出:['one', 'two', 'three', 'four']
场景5:查找所有IP地址
text = "Server 192.168.1.1 is up, and server 10.0.0.1 is down."
ips = re.findall(r'\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}', text)
print(ips) # 输出:['192.168.1.1', '10.0.0.1']
第六部分:常见Bug及解决方案
Bug1:模式不匹配
错误信息:re.error: unbalanced parenthesis
# 错误的正则表达式
pattern = r'(abc'
text = "abc"
match = re.search(pattern, text)# 解决方案
pattern = r'(abc)' # 添加闭合的括号
Bug2:特殊字符未转义
错误信息:re.error: special characters not allowed
# 错误的正则表达式
pattern = r'new line\n'
text = "new line\n"
match = re.search(pattern, text)# 解决方案
pattern = r'new line\\n' # 转义特殊字符
Bug3:模式匹配失败
错误信息:AttributeError: 'NoneType' object has no attribute 'group'
# 错误的代码
pattern = r'no match'
text = "some text"
match = re.search(pattern, text)
print(match.group()) # 没有匹配时,match为None# 解决方案
if match:print(match.group())
else:print("No match found")
第七部分:总结
re
库是Python中处理字符串的强大工具,它通过正则表达式提供了灵活的文本匹配和处理能力。通过本文的介绍,你已经了解了re
库的基本使用方法和一些实际应用场景。掌握re
库,将使你在文本处理方面更加得心应手。记住,正则表达式的强大之处在于其灵活性和表达能力,但也需要仔细编写和测试以避免常见的错误。
如果你觉得文章还不错,请大家 点赞、分享、留言 下,因为这将是我持续输出更多优质文章的最强动力!