这里写目录标题
- 使用现有数据运行模拟器
- 初始化数据
- 格式化数据用于训练和保存
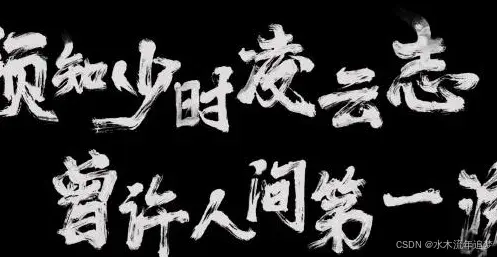
使用现有数据运行模拟器
考虑输入数据已经存在并且已有因果结构的情况。我们希望根据这些数据模拟治疗分配和结果。
初始化数据
首先我们将所需数据加载到pandas DataFrame中:
import pandas as pd
from causallib.datasets import load_nhefs
from causallib.datasets import CausalSimulator
from causallib.datasets import generate_random_topology
data = load_nhefs()
X_given = data.X
假设我们要创建三个额外变量:协变量、治疗和结果。这样很难硬编码很多变量,所以我们使用随机拓扑生成器:
topology, var_types = generate_random_topology(n_covariates=1, p=0.4,n_treatments=1, n_outcomes=1,given_vars=X_given.columns)
现在我们根据变量拓扑创建模拟器:
outcome_types = "categorical"
link_types = ['linear'] * len(var_types)
prob_categories = pd.Series(data=[[0.5, 0.5] if typ in ["treatment", "outcome"] else None for typ in var_types],index=var_types.index)
treatment_methods = "gaussian"
snr = 0.9
treatment_importance = 0.8
effect_sizes = None
sim = CausalSimulator(topology=topology.values, prob_categories=prob_categories,link_types=link_types, snr=snr, var_types=var_types,treatment_importances=treatment_importance,outcome_types=outcome_types,treatment_methods=treatment_methods,effect_sizes=effect_sizes)
现在为了根据给定数据生成数据,我们需要指定:
X, prop, y = sim.generate_data(X_given=X_given)
格式化数据用于训练和保存
既然我们已经生成了一些数据,我们可以将其格式化以便更容易训练和验证:
observed_set, validation_set = sim.format_for_training(X, prop, y)
可观测集是可观测数据集(不包括隐藏变量)验证集用于验证目的——包含反事实、样本的治疗分配和倾向。你可以将数据集保存为csv:
covariates = observed_set.loc[:, observed_set.columns.str.startswith("x_")]
print(covariates.shape)
covariates.head()
(1566, 19)
treatment_outcome = observed_set.loc[:, (observed_set.columns.str.startswith("t_") |observed_set.columns.str.startswith("y_"))]
print(treatment_outcome.shape)
treatment_outcome.head()
(1566, 2)
print(validation_set.shape)
validation_set.head()
(1566, 5)