在第i个位置插入 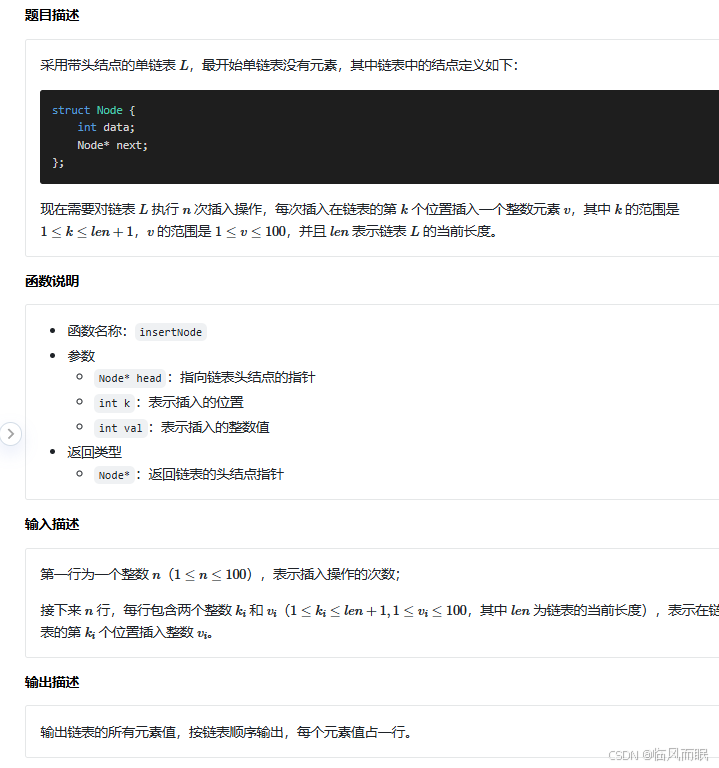
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <limits.h>
#include <math.h>
#include <stdbool.h>typedef struct Node {int data;struct Node* next;
} Node;Node* newNode(int data);/* 关键函数 */
void insertNode(Node* head, int k, int val) {// 在第k个位置插入正数value// 那么就要找到第k-1个位置Node* p = head;Node* v = newNode(val);for(int i = 1; i <= k-1; i++){ p = p-> next;}//此时要先接入到第k个元素v->next = p->next;p->next = v;}Node* newNode(int data) {Node* node = (Node*)malloc(sizeof(Node));node -> data = data;node -> next = NULL;return node;
}int main() {int n;scanf("%d", &n);Node* head = newNode(-1);for (int i = 0; i < n; i++) {int k, data;scanf("%d%d", &k, &data);insertNode(head, k, data);}Node* temp = head -> next;while (temp != NULL) {printf("%d\n", temp -> data);temp = temp -> next;}return 0;
}
删除
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <limits.h>
#include <math.h>
#include <stdbool.h>typedef struct Node {int data;struct Node* next;
} Node;Node* newNode(int data);/* 关键函数 */
void removeNode(Node* head, int k) {// 删除第k个Node* p = head;for(int i = 1; i <= k-1; i++){p = p-> next;}Node*q = p-> next;p->next = q->next;free(q);
}Node* newNode(int data) {Node* node = (Node*)malloc(sizeof(Node));node -> data = data;node -> next = NULL;return node;
}int main() {int n;scanf("%d", &n);Node* L = newNode(-1);Node* last = L;for (int i = 0; i < n; i++) {int data;scanf("%d", &data);last -> next = newNode(data);last = last -> next;}int k;scanf("%d", &k);removeNode(L, k);Node* temp = L -> next;while (temp != NULL) {printf("%d\n", temp -> data);temp = temp -> next;}return 0;
}
原地逆转
头插法
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <limits.h>
#include <math.h>
#include <stdbool.h>typedef struct Node {int data;struct Node* next;
} Node;Node* newNode(int data);
/* 关键函数 */
void reverseList(Node* head) {Node * current = head->next;head -> next = NULL;while(current!=NULL){Node* nextNode = current->next;current->next = head->next; //这一行和下一行就是头插head->next = current;current = nextNode; //这一行和第一行是不断往后移动}
}Node* newNode(int data) {Node* node = (Node*)malloc(sizeof(Node));node -> data = data;node -> next = NULL;return node;
}int main() {int n;scanf("%d", &n);Node* L = newNode(-1);Node* last = L;for (int i = 0; i < n; i++) {int data;scanf("%d", &data);last -> next = newNode(data);last = last -> next;}reverseList(L);Node* temp = L -> next;printf("%d", temp -> data);temp = temp -> next;while (temp != NULL) {printf(" %d", temp -> data);temp = temp -> next;}return 0;
}