红黑树的概念
红黑树也是一颗搜索二叉树,它每个节点的颜色不是红色就是黑色,所以叫做红黑树
C++:二叉搜索树-CSDN博客
它可以确保没有一条路径比其他路径长出2倍,因此它接近平衡
红黑树的规则
- 每个节点不是红色就是黑色
- 根节点是黑色
- 如果⼀个结点是红色的,则它的两个孩⼦结点必须是⿊色的,也就是说任意⼀条路径不会有连续的红色结点
- 对于任意一个节点,该节点到NULL节点的路径上均包含相同数量的黑色节点
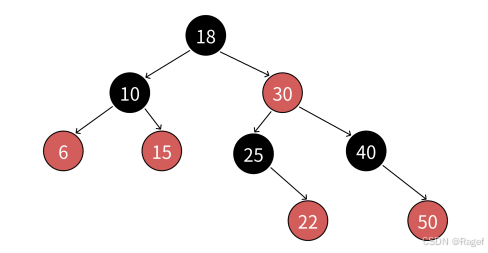
根据红黑树的规则,它之所以能做到没有一条路径比其他路径长出2倍是因为规则3和4
最短的节点路径上的颜色就是全黑,而相对最长的路径就是全部一黑一红,所以最长只能是两倍
红黑树和AVL树相比,红黑树的整体高度会偏高一些,但是由于它的特性,它旋转的次数会相较于AVL树的旋转次数少,并且它们的高度差也只差很小的常数,这高度差对于CPU的运行速度来讲都没有区别,所以红黑树的效率也是极高的
红黑树的实现
红黑树的结构
enum Colour
{RED,BLACK
};template<class K, class V>
struct RBTreeNode
{pair<K, V> _kv;RBTreeNode<K, V>* _left;RBTreeNode<K, V>* _right;RBTreeNode<K, V>* _parent;Colour _col;RBTreeNode(const pair<K, V>& kv):_kv(kv), _left(nullptr), _right(nullptr), _parent(nullptr){}
};template<class K, class V>
class RBTree
{typedef RBTreeNode<K, V> Node;
public:
private:Node* _root = nullptr;
};
首先用了一个枚举的类型枚举了红色和黑色两种颜色,当然也可以使用数字来表示
这种枚举好处在于在调试的时候是可以直观的看见RED和BLACK的,而不是数字等
红黑树的节点和AVL树一样都需要三叉链,值用pair来存储
红黑树类里面只需要一个root节点的指针即可
红黑树的插入
bool Insert(const pair<K, V>& kv)
{if (_root == nullptr){_root = new Node(kv);_root->_col = BLACK;return true;}Node* parent = nullptr;Node* cur = _root;while (cur){if (cur->_kv.first < kv.first){parent = cur;cur = cur->_right;}else if (cur->_kv.first > kv.first){parent = cur;cur = cur->_left;}else{return false;}}cur = new Node(kv);cur->_col = RED;if (parent->_kv.first < kv.first){parent->_right = cur;}else{parent->_left = cur;}cur->_parent = parent;while (parent && parent->_col == RED){Node* grandfather = parent->_parent;if (grandfather->_left == parent){Node* uncle = grandfather->_right;if (uncle && uncle->_col == RED){// 变色parent->_col = uncle->_col = BLACK;grandfather->_col = RED;// 继续向上处理cur = grandfather;parent = cur->_parent;}else{if (cur == parent->_left){RotateR(grandfather);parent->_col = BLACK;grandfather->_col = RED;}else{RotateL(parent);RotateR(grandfather);cur->_col = BLACK;parent->_col = grandfather->_col = RED; // 1}break;}}else{Node* uncle = grandfather->_left;if (uncle && uncle->_col == RED){// 变色parent->_col = uncle->_col = BLACK;grandfather->_col = RED;// 继续向上处理cur = grandfather;parent = cur->_parent;}else{if (cur == parent->_right){RotateL(grandfather);parent->_col = BLACK;grandfather->_col = RED;}else{RotateR(parent);RotateL(grandfather);cur->_col = BLACK;parent->_col = grandfather->_col = RED; // 1}break;}}}// 确保根节点颜色是黑色_root->_col = BLACK;return true;
}
前面的插入逻辑和搜索二叉树、AVL树的插入逻辑都一样,毕竟它们都是同一个规则
只需要注意的是插入的如果是根节点,则需要插入的是黑色的节点(红黑树的规则)
其余的只需要插入红色的节点即可
为什么插入的新节点是红色节点而不是黑色节点
根据红黑树的四个规则
- 每个节点不是红色就是黑色
- 根节点是黑色
如果⼀个结点是红色的,则它的两个孩⼦结点必须是⿊色的,也就是说任意⼀条路径不会有连续的红色结点 对于任意一个节点,该节点到NULL节点的路径上均包含相同数量的黑色节点如果我们插入的是红色节点,那么我们需要注意的是规则3,不能有相邻的红色节点如果我们插入的是黑色节点,那么我们需要注意的是规则4,每条路径需要有相同数量的黑色节点从调整的方向来看,我们即使违反了规则3那也只是一小部分的红黑树规则遭到破坏但如果我们违反的是规则4的话,每一条路径都会需要调整所以我们插入的是红色节点
下面大多的代码就是维护红黑树的结构
因为我们插入的是红色节点,所以我们只需要判断它的parent节点的颜色是否为红色
当parent节点在grandparent节点的左边时
若uncle节点也为红色,我们可以把grandparent节点变红,parent和uncle节点变黑,这样我们的规则3的问题就解决了,规则4也没有触犯到
我们这个语句是需要循环往上执行的,因为当我们把grandfather变红后,保不齐它的father就是红色,这样又会违反规则3,所以我们需要循环处理
若uncle不如我们所料,那我们就需要旋转处理了
若是cur在parent的左边上图所示的情况,无论我们怎么改变颜色都解决不了问题,这时候我们就需要引入AVL中写过的旋转来解决
只需要对grandfather进行右旋+变色即可
旋转完后需要注意颜色不能违反规则,让parent变成黑色(它有可能是根节点),grandfather和cur变成红色即可
若是cur在parent的右边如上图所示,这样我们只进行单旋是解决不了问题的,很明显p和c偏向右,需要左单选,而从整体的g看来是需要右单旋的,这时候就只需要双旋+变色即可
先对p左单旋,解决它偏向右的问题,然后再对g右单旋,这时候整体就平衡了
这时候只需要和上面一样调整变色即可
剩下下面的代码的逻辑完全和上面的一样
和上面的区别就是上面的parent是在grandparent的左边,而下面的parent是在grandparent的右边,只有方向上面的区别
最后需要确保根节点的颜色是黑色,因为我们在调整的过程中可能会无意中把根节点的颜色变成红色,在各个 if 语句中解决起来相对麻烦一些,所以我们在最后修改root的颜色为黑色就解决了
红黑树的查找
Node* Find(const K& key)
{Node* cur = _root;while (cur){if (cur->_kv.first < key){cur = cur->_right;}else if (cur->_kv.first > key){cur = cur->_left;}else{return cur;}}return nullptr;
}
查找逻辑和搜索二叉树一致,搜索效率为O(logN)
红黑树的验证
bool Check(Node* root, int blackNum, const int refNum)
{if (root == nullptr){if (blackNum != refNum){cout << "存在黑色结点的数量不相等的路径" << endl;return false;}return true;}if (root->_col == RED && root->_parent->_col == RED){cout << root->_kv.first << "存在连续的红色结点" << endlC;return false;}if (root->_col == BLACK){blackNum++;}return Check(root->_left, blackNum, refNum) && Check(root->_right, blackNum, refNum);
}bool IsBalance()
{if (_root == nullptr)return true;if (_root->_col == RED)return false;int refNum = 0;Node* cur = _root;while (cur){if (cur->_col == BLACK)refNum++;cur = cur->_left;}return Check(_root, 0, refNum);
}
Check函数的第一个参数为根节点,第二个参数需要记录黑色节点的数量,第三个参数为黑色节点的其中一个参考值
这里的参考值是在IsBalance中传递的,这个参考值是随机选取一条路径记录黑色节点的数量,接着用这个参考值在Check函数中检查每一条路径的黑色节点与之对比,若不相等则说明存在黑色节点的数量不相等的路径
判断连续相同的红色节点
如果我们从parent来判断它的左右孩子是否为红色就比较麻烦
我们可以直接判断若当前节点颜色为红色,并且它的parent也为红色,则说明存在连续的红色节点
完整代码
#pragma onceenum Colour
{RED,BLACK
};template<class K, class V>
struct RBTreeNode
{pair<K, V> _kv;RBTreeNode<K, V>* _left;RBTreeNode<K, V>* _right;RBTreeNode<K, V>* _parent;Colour _col;RBTreeNode(const pair<K, V>& kv):_kv(kv), _left(nullptr), _right(nullptr), _parent(nullptr){}
};template<class K, class V>
class RBTree
{typedef RBTreeNode<K, V> Node;
public:bool Insert(const pair<K, V>& kv){if (_root == nullptr){_root = new Node(kv);_root->_col = BLACK;return true;}Node* parent = nullptr;Node* cur = _root;while (cur){if (cur->_kv.first < kv.first){parent = cur;cur = cur->_right;}else if (cur->_kv.first > kv.first){parent = cur;cur = cur->_left;}else{return false;}}cur = new Node(kv);cur->_col = RED;if (parent->_kv.first < kv.first){parent->_right = cur;}else{parent->_left = cur;}cur->_parent = parent;while (parent && parent->_col == RED){Node* grandfather = parent->_parent;if (grandfather->_left == parent){Node* uncle = grandfather->_right;if (uncle && uncle->_col == RED){// 变色parent->_col = uncle->_col = BLACK;grandfather->_col = RED;// 继续向上处理cur = grandfather;parent = cur->_parent;}else{if (cur == parent->_left){RotateR(grandfather);parent->_col = BLACK;grandfather->_col = RED;}else{RotateL(parent);RotateR(grandfather);cur->_col = BLACK;parent->_col = grandfather->_col = RED;}break;}}else{Node* uncle = grandfather->_left;if (uncle && uncle->_col == RED){// 变色parent->_col = uncle->_col = BLACK;grandfather->_col = RED;// 继续向上处理cur = grandfather;parent = cur->_parent;}else{if (cur == parent->_right){RotateL(grandfather);parent->_col = BLACK;grandfather->_col = RED;}else{RotateR(parent);RotateL(grandfather);cur->_col = BLACK;parent->_col = grandfather->_col = RED;}break;}}}// 确保根节点颜色是黑色_root->_col = BLACK;return true;}void RotateR(Node* parent){Node* subL = parent->_left;Node* subLR = subL->_right;parent->_left = subLR;if (subLR)subLR->_parent = parent;Node* parentParent = parent->_parent;subL->_right = parent;parent->_parent = subL;if (parent == _root){_root = subL;subL->_parent = nullptr;}else{if (parentParent->_left == parent){parentParent->_left = subL;}else{parentParent->_right = subL;}subL->_parent = parentParent;}}void RotateL(Node* parent){Node* subR = parent->_right;Node* subRL = subR->_left;parent->_right = subRL;if (subRL)subRL->_parent = parent;Node* parentParent = parent->_parent;subR->_left = parent;parent->_parent = subR;if (parentParent == nullptr){_root = subR;subR->_parent = nullptr;}else{if (parent == parentParent->_left){parentParent->_left = subR;}else{parentParent->_right = subR;}subR->_parent = parentParent;}}void InOrder(){_InOrder(_root);cout << endl;}int Height(){return _Height(_root);}int Size(){return _Size(_root);}Node* Find(const K& key){Node* cur = _root;while (cur){if (cur->_kv.first < key){cur = cur->_right;}else if (cur->_kv.first > key){cur = cur->_left;}else{return cur;}}return nullptr;}bool IsBalance(){if (_root == nullptr)return true;if (_root->_col == RED)return false;int refNum = 0;Node* cur = _root;while (cur){if (cur->_col == BLACK)refNum++;cur = cur->_left;}return Check(_root, 0, refNum);}
private:bool Check(Node* root, int blackNum, const int refNum){if (root == nullptr){if (blackNum != refNum){cout << "存在黑色结点的数量不相等的路径" << endl;return false;}return true;}if (root->_col == RED && root->_parent->_col == RED){cout << root->_kv.first << "存在连续的红色结点" << endlC;return false;}if (root->_col == BLACK){blackNum++;}return Check(root->_left, blackNum, refNum) && Check(root->_right, blackNum, refNum);}void _InOrder(Node* root){if (root == nullptr){return;}_InOrder(root->_left);cout << root->_kv.first << ":" << root->_kv.second << endl;_InOrder(root->_right);}int _Height(Node* root){if (root == nullptr)return 0;int leftHeight = _Height(root->_left);int rightHeight = _Height(root->_right);return leftHeight > rightHeight ? leftHeight + 1 : rightHeight + 1;}int _Size(Node* root){if (root == nullptr)return 0;return _Size(root->_left) + _Size(root->_right) + 1;}private:Node* _root = nullptr;
};
完